Introduction The DS1WM1-Wire bus master generates 1-Wire timing and control signals internally, without the CPU generating bit-by-bit control timing. This control function enables system programmers to use API functions for program development. The DS1WM API is written in ANSI C language and can be used by a variety of microprocessor platforms that support ANSI C. The following routine describes how to identify, select, and communicate with 1-Wire slave devices on the network.
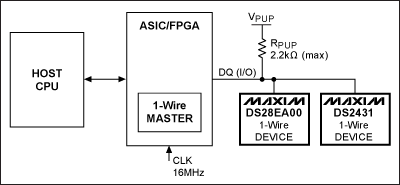
Figure 1. Example circuit of a 1-Wire network bus
The circuit configuration shown in Figure 1 applies to all the following sample programs. The host CPU uses the DS1WM to communicate with the 1-Wire digital temperature sensor DS28EA00 with link mode and GPIO and the 1-Wire 1Kb EEPROM DS2431. The sample program focuses on demonstrating the API, not the functionality of the slave device. The 16MHz system clock provides 1-Wire master timing through the CLK pin. The port pins of the 1-Wire master need to be mapped to the pins of the application microprocessor. The API file DS1WM.h contains the pin mapping function. The MaxNumberDevices macro in the API header file must be changed to the actual maximum possible number of devices. The ReadByteFromRegister and WriteByte2Register functions in the DS1WM.c API file also need to be modified to properly trigger the microprocessor port. The typical value of VPUP voltage is 5.0V, and RPUP is not greater than 2.2kΩ. Overview Through the Maxim Support Center, you can apply for free DS1WM and API. The DS1WM codes for initialization and 1-Wire reset control are discussed below. An example is also provided to demonstrate how to use the DS1WM ROM search engine to determine the unique registration code (ROM ID) of each device. Using the stored registration code and family code, each 1-Wire slave device can be identified and accessed by matching ROM commands. For a list of family codes, see Application Note 155: "1-Wire® Software Resource Guide". The DS28EA00 example demonstrates how to perform temperature conversion. The second example shows two ways to read and write the DS2431 scratchpad at high speed.
The basic operation of the bus master microprocessor initializes the DS1WM by triggering the reset pin, and the API interface performs all 1-Wire communications. The sample program works by calling the DS1WM API function. Because the microprocessor code is changed when responding to an interrupt, polling is used instead of interrupt to communicate in subsequent examples.
The 1-Wire operation shown in Table 1 is used in the subsequent sample program.
Table 1. 1-Wire operation
OperaTIon * | DescripTIon |
OWReset | Sends the 1-Wire reset sTImulus and checks for the pulses of 1-Wire slave devices that are present on the bus. |
OWWriteByte / OWReadByte | Sends or receives a single byte of data from the 1-Wire bus. |
OWWriteBytes / OWReadBytes | Sends or receives mulTIple bytes of data from the 1-Wire bus. |
OWSearch | Performs the 1-Wire Search Algorithm (see application note 187, "1-Wire Search Algorithm"). |
OverdriveEnable | Sets the 1-Wire communication speed for the DS1WM to overdrive. Note that this only changes the communication speed of the DS1WM; the 1-Wire slave device must be instructed to make the switch when going from normal to overdrive. The 1-Wire slave will always revert to standard speed when it encounters a standard-speed 1-Wire reset. |
OverdriveDisable | Sets the 1-Wire communication speed for the DS1WM to standard. Note that this only changes the communication speed of the DS1WM; a standard-speed 1-Wire reset is required for slave devices to exit overdrive. |
MatchROM | Selects device by issuing the Match ROM command followed by the 64-bit ROM ID selected. |
SetClockFrequency | Sets the clock frequency for the DS1WM. |
InterruptEnableRegisterWrite | Writes a single byte of data to the DS1WM Interrupt Enable register. |
InterruptRegisterRead | Reads a single byte of data from the DS1WM Interrupt register. |
ReceiveBufferRead | Reads a single byte of data from the DS1WM Receive Buffer register. |
Initialization starts DS1WM by initializing the host and providing the correct 1-Wire bus timing. The host passes the value 16 (representing the frequency value) to the SetClockFrequency function, and writes 0x90h to the clock divider register (see the DS1WM data sheet). The interrupt enable register is set to 0x00h to prevent the INTR pin from generating an interrupt. At power-on, the receive buffer may contain invalid values, so it is best to clean the receive buffer before sending a 1-Wire command. Reading the interrupt register and the receive buffer at startup will clear each bit.
// ------------------------------------------------ -------------------------------------------------- -// Start of initialization example SetClockFrequency (16); // Set clock frequency to 16MHz (power-on default) InterruptEnableRegisterWrite (0x00); // Clear interrupts // Flush receive buffer InterruptRegisterData = InterruptRegisterRead (); ReceiveBufferRead (); // End of initialization example // ------------------------------------------ -------------------------------------------------- -------- After the 1-Wire reset (OWReset API function) is initialized, the host must determine whether a device is connected to the 1-Wire bus. The host calls the OWReset function to achieve this purpose. If a pulse is detected in the device, the function returns 1; if no device is detected or an error is sent, the function returns 0. If the return value is 0, you need to check the ErrorStatus variable to determine the fault status. The 1-Wire reset has higher priority than all 1-Wire commands (for example, Match ROM, Skip ROM, Read ROM), except when calling the OWSearch function, which has its own reset. Software designers should add appropriate fault handling code to the program, which can trigger the execution of these codes when a fault occurs. The ErrorStatus value means that one of the following errors has occurred: no online detection; no device detected; there is a 1-Wire short circuit; the 1-Wire bus is always low.
Result = OWReset (); if (! Result) {switch (ErrorStatus) {case -1: // DS1WM did not recognize 1-Wire reset (PD = 0) // To d add your error code here break; case -2 : // No device found (PDR = 1) // To d add your error code here break; case -7: // 1-Wire IO is shorted (OW_SHORT = 1) // To d add your error code here break; case -8: // 1-Wire IO is shorted (OW_LOW = 1) // To d add your error code here break;}} Use the ROM search engine to determine that each 1-Wire device on the ROM ID bus must be recognized . The unique 64-bit ROM ID stored in each 1-Wire device is used to select each device and identify the device type based on the family code. To reduce the complexity of the routine, the 8-bit family code is used to identify the DS28EA00 and DS2431.
Note: If more than one device type is connected to the 1-Wire bus (for example, with the same family code), subsequent methods cannot identify the device. Without identifying multiple 1-Wire devices on the bus, it is not possible to send functional commands to specific 1-Wire devices. If two or more similar devices are used, a specific device must be accessed correctly through a look-up table.
Call the OWSearch function to identify all 1-Wire devices on the bus. This function arranges the 8-byte ROM IDs in a row. There is no need to call the OWReset function during this period, because this function is already included in the OWSearch function. The exception of the OWSearch function includes the OWReset function because its search algorithm has an iterative process. The return value of the OWSearch function is the number of 1-Wire devices found, and each 64-bit ROM ID is written into a two-dimensional array called ROMCodes.
The following example only demonstrates the case of calling OWSearch once, so subsequent devices connected to the 1-Wire bus will not be recognized by this code. After the OWSearch function is successfully executed, the number of searched devices will be returned. If the search fails, the ErrorStatus variable will return an error value, which includes the fault condition that generated OWReset. After the search is successful, the ROMCodes array is traversed, and the array index of each device is saved. This device indexing method is realized by comparing the family code of each device found. Then call the array index to communicate with the specific 1-Wire device found. This is equivalent to creating a simple linked list (Note: You can operate through pointers).
// ------------------------------------------------ -------------------------------------------------- -// Start of DS1WM search ROM accelerator example // Devices on the 1-Wire IO are: // DS28EA00 Family Code = 42h (6900000004E8C842) // DS2431 Famliy Code = 2Dh (5A0000000FDE052D) // Find all devices on 1- Wire line and populate ROMCodes array Result = OWSearch (ROMCodes); // Returns number of devices found if successful // Set number of 1-Wire devices found if (Result) NumberOfDevices = Result; if (! Result) {switch (ErrorStatus) {case -1: // DS1WM did not recognize 1-Wire Reset (PD = 0) // To d add your error code here break; case -2: // No device found (PDR = 1) // To d add your error code here break; case -7: // 1-Wire IO is shorted (OW_SHORT = 1) // To d add your error code here break; case -8: // 1-Wire IO is shorted (OW_LOW = 1 ) // To d add your error code here break; case -9: // Invalid CRC for device // To d add your error code here break; case -10: // ROMCodes array too small (Edit MaxNumberDevices in DS1WM. h) // To d add your error code here break;}} // Note: This function is intended to be used when there is only one device with the same // Family Code present on the line for (i = 0; i
The OWWriteByte and OWReadByte API functions are used to generate memory commands (for example, Write / Copy / Read Scratchpad commands). DS1WM sets the temperature alarm and resolution by sending the Write Scratchpad command (0x4Eh) and the subsequent high temperature, low temperature, and configuration register settings. After the above operation is completed, send the OWReset and Match ROM command, ROM ID and Copy Scratchpad command (0x48h) (copy the contents of the scratchpad to the register memory) to complete the temperature resolution setting. A 10ms delay must be added to the CPU host to complete the copy operation. Considering the differences in microprocessor delay subroutines, the API only provides comment pseudocode.
After the copy operation is completed, execute OWReset again, and then send the Match ROM, ROM ID, and conversion temperature command (0x44h). Need to increase the delay of 100ms to complete the temperature conversion. Finally, send OWReset and Match ROM, ROM ID and Read Scratchpad commands (0xBEh) to read two bytes of temperature data. It should be noted that when choosing DS28EA00, you must always follow the command format of sending the Match ROM command and ROM ID after OWReset. When there is only one device on the bus, the Skip ROM command can be used (that is, no Search ROM is required).
// ------------------------------------------------ -------------------------------------------------- -// Start of DS28EA00 example Result = OWReset (); if (! Result) {switch (ErrorStatus) {case -1: // DS1WM did not recognize 1-Wire reset (PD = 0) // To d add your error code here break; case -2: // No device found (PDR = 1) // To d add your error code here break; case -7: // 1-Wire IO is shorted (OW_SHORT = 1) // To d add your error code here break; case -8: // 1-Wire IO is shorted (OW_LOW = 1) // To d add your error code here break;}} // Set temperature resolution Result = MatchROM (ROMCodes, DS28EA00 ); // Select device Result = OWWriteByte (0x4E); // Issue Write Scratchpad command Result = OWWriteByte (0x00); // TH register data Result = OWWriteByte (0x00); // TL degister data Result = OWWriteByte (0x1F); // Config. Reg. Data (set 9-bit temp. Resolution) OWReset (); // Error code removed for conciseness MatchROM (ROMCodes, DS28EA00); // Select device OWWriteByte (0x48); // Issue Copy Scratchpad command / / To d add microproces sor-specific code delay to allow copy to complete // Delay (10MS); // Psuedo code OWReset (); // 1-Wire reset MatchROM (ROMCodes, DS28EA00); // Select device OWWriteByte (0x44); // Issue Convert Temperature command // To d add microprocessor-specific code delay to allow temperature conversion to complete // Delay (100MS); // Psuedo code // Read temperature results from scratchpad OWReset (); // 1-Wire reset MatchROM (ROMCodes , DS28EA00); // Select device OWWriteByte (0xBE); // Issue Read Scratchpad command TempLSB = OWReadByte (); // Read byte TempMSB = OWReadByte (); // Read byte // End of DS28EA00 example // --- -------------------------------------------------- ----------------------------------------------- Using the DS1WM API DS2431 in function-controlled high-speed mode This example uses the previously searched ROM ID to communicate with the DS2431. After OWReset, send the Overdrive Skip ROM command (0x3Ch) to put all 1-Wire devices that support high-speed mode into high-speed mode. Call the OverdriveEnable function to activate the DS1WM high-speed timing. At this time, all 1-Wire devices work in high-speed mode. For 1-Wire timing in standard rate mode and high-speed mode, see Application Note 126: "1-Wire® Communication in Software".
Using Target Address TA1 and TA2 Variables In the first example method, the variables TA1 and TA2 are set to the memory addresses expected by the DS2431. Send OWReset, followed by Match ROM, DS2431 ROM ID, Write Scratchpad command (0x0Fh), target address 1 & 2, and 8 bytes of data to be written to DS2431 64-bit scratchpad. It is recommended to perform readback and CRC16 check operations. For a detailed discussion of CRC16, please refer to Application Note 27: "Understanding and Using Cyclic Redundancy Check (CRC) in Maxim iButton® Products".
In the second example method, OWWriteBytes and OWReadBytes call two new API functions: OWWriteBytes and OWReadBytes. These two API functions simplify the read and write operations of a large amount of data to the scratchpad.
The method of writing to the scratchpad is: setting the target address 1 & 2, writing data to the WriteBytes array, executing OWReset, and then sending the Match ROM, DS2431 ROM ID, Write Scratchpad command (0x0Fh), the target address 1 & 2, using the OWWriteBytes function Write all 10 bytes saved in WriteBytes, read back CRC16 and check CRC16.
The method of reading the scratchpad is: execute OWReset, send Match ROM, DS2431 ROM ID, Read Scratchpad command (0xAAh), use the OWReadBytes function to read all 13 bytes (TA1, TA2, ES, CRC16 & 8 byte data) And save to ReadBytes.
Call the API function OverdriveDisable, and then send the standard OWReset to exit the high-speed modulus and return all devices to the standard rate.
// ------------------------------------------------ -------------------------------------------------- -// Start of DS2431 example Result = OWReset (); // Error code removed for conciseness Result = OWWriteByte (0x3C); // Overdrive Skip ROM (all devices are now in overdrive) OverdriveEnable (); // Enable Overdrive Mode // Write scratchpad with data // First method TA1 = 0x00; TA2 = 0x00; Result = OWReset (); // 1-Wire reset Result = MatchROM (ROMCodes, DS2431); // Select device Result = OWWriteByte (0x0F); // Issue Write Scratchpad command Result = OWWriteByte (TA1); // Send target address 1 (TA1) Result = OWWriteByte (TA2); // Send target address 2 (TA2) // Write 8 Bytes of Data Result = OWWriteByte (0x11 ); // Send Data Byte for all Result = OWWriteByte (0x22); Result = OWWriteByte (0x33); Result = OWWriteByte (0x44); Result = OWWriteByte (0x55); Result = OWWriteByte (0x66); Result = OWWriteByte (0x77) ; Result = OWWriteByte (0x88); // It is recommended that the CRC16 be read back and verified // CRC16 code was left o ut for conciseness Result = OWReset (); // 1-Wire Reset Result = MatchROM (ROMCodes, DS2431); // Select device Result = OWWriteByte (0xAA); // Issue Read Scratchpad command Result = OWReadByte (); // Read TA1 if (Result! = TA1) {// To d Add your error code here} Result = OWReadByte (); // Read TA2 if (Result! = TA2) {// To d Add your error code here} ES = OWReadByte (); // Read ES // To d add your error code after reads Result = OWReadByte (); // Read Data Byte (0x11) Result = OWReadByte (); // Read Data Byte (0x22) Result = OWReadByte () ; // Read Data Byte (0x33) Result = OWReadByte (); // Read Data Byte (0x44) Result = OWReadByte (); // Read Data Byte (0x55) Result = OWReadByte (); // Read Data Byte (0x66 ) Result = OWReadByte (); // Read Data Byte (0x77) Result = OWReadByte (); // Read Data Byte (0x88) // It is recommended that the CRC16 be read back and verified // CRC16 code was left out for conciseness // Second method TA1 = 0x00; TA2 = 0x00; WriteBytes [0] = TA1; WriteBytes [1] = TA2; for (i = 2; i <10; i ++) {WriteBytes [i ] = i;} Result = OWReset (); // 1-Wire reset Result = MatchROM (ROMCodes, DS2431); // Select device Result = OWWriteByte (0x0F); // Issue Write Scratchpad command // Write 10 bytes of data (TA1, TA2 & 8 bytes of data) OWWriteBytes (WriteBytes, 10); // Write data bytes // It is recommended that the CRC16 be read back and verified // CRC16 code was left out for conciseness Result = OWReset (); // 1-Wire reset Result = MatchROM (ROMCodes, DS2431); // Select device Result = OWWriteByte (0xAA); // Issue Read Scratchpad command // Read 13 bytes of data (TA1, TA2, ES, CRC16 & 8 bytes of data) OWReadBytes (ReadBytes, 13); // Read data bytes // It is recommended that the CRC16 be read back and verified // CRC16 code was left out for conciseness // Exit overdrive OverdriveDisable (); Result = OWReset () ; // Std. Reset issued (all devices are now in standard speed) // End of DS2431 example // -------------------------- -------------------------------------------------- ------------------------ Conclusion This application note gives DS1WM exemplary API functions of the control, the host CPU need not produce 1-Wire timing. The user should now have a certain understanding of the general API functions for selecting and accessing multiple 1-Wire devices on the bus. This article gives examples of control and access to the DS28EA00 and DS2431 bus example devices. It also demonstrates high-speed mode and single-byte / multi-byte data read and write operations.
We offer Industry's leading lipo cells from Panasonic, Sanyo, Samsung, ATL, Wanma, HYB, DLG, BAK, as well as sourcing low cost cells from other small size Lipo cell factories. There are regular lipo cells with discharge rate below 1C, and high discharge rate lipo cells up to 40C.
lithium polymer battery, Lithium Ion Polymer Battery, polymer battery, lithium polymer, 3.7 v Lipo Battery , lipo, batterie lithium polymère, li ion li polymer, batterie lipo, li po, lithium ion vs lithium polymer.
3 v battery, 3 volt lipo battery, 3v lithium, 3.2 v lipo battery,
3.2 volt lithium battery, 3.2v Lithium Ion Battery, 3.3 v battery pack,
3.3v lipo battery, 3.5 volt Rechargeable Batteries,
3.6 Lithium Ion polymer battery, 3.6 v LiPo, 3.6 volt LIP,
3.7 volt single cell battery pack, 3.7v 1s Lipo,
3.8 lipo cell, 3.8 v Lipo Rechargeable Battery, 3.8v rechargeable batteries,
3.7 Li-poly, 3.7 lithium-poly, 3.7 v battery cell.
4 v li battery, 4.2 v lipo battery, 4.2 volt lithium ion polymer rechargeable battery.
Lipo Battery
Lipo Battery,Lithium Ion Polymer Battery,Lipo Rechargeable Battery,Lithium-Poly Battery
Asarke Industry Co., Limited , https://www.asarke-industry.com